
With the above code, the element would be inserted just before the last element of the original array. In such cases, the desired behavior may differ from one project to another. One problem that can arise with the use of this technique is that the element may not exist in the array at all. People.splice(index, 0, "Rhonda", "Kerry") Once you have the index, you can use any of the first two methods in this post to insert the new elements into the original array. This can be done using the Array.indexOf() method. In such cases, you can start by first finding the index of the element after which you want to insert the new elements. This is slightly different from inserting elements at a certain index. Sometimes you might have to insert an element after another one with a specific value. Insert One or Multiple Elements Before a Specific Element in an Array However, if that is what you intend to do, using push() might be more appropriate than using splice(). The only limitation of this technique is that the elements can only be inserted at the end of the array. Otherwise, they will be inserted as a single array.
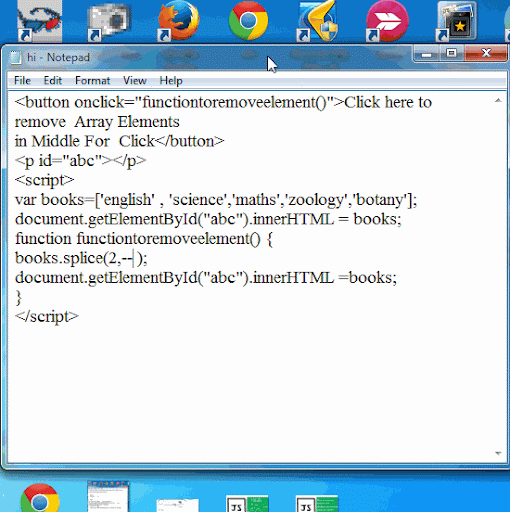
Keep in mind that you will have to specify all the elements that you want to insert into the array in separate arguments. You can simply use Array.push() and specify the elements that you want to append. If you are only planning to insert one or multiple elements at the end of an array, you don’t have to use any complicated methods.
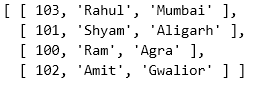
Insert One or Multiple Elements at the End of an Array Using Array.push() The second slice() method returns all elements from the given index up to the end of the original array. The first slice() method returns all elements from the beginning of the array up to index - 1 where we want to insert the new elements. In the above example, we have used the spread operator along with two calls to the slice() method in order to create a new array with all the elements inserted into it. Here is an example which shows the spread operator in action: One advantage of using this technique is that you don’t have to mutate the original array. The slice() method returns a shallow copy of a portion of the calling array into a new array object selected from begin to end (end not included). We can use this operator along with the Array.slice() method to insert one or more elements into an array at any given position. The spread operator in ES6, which is written as three dots (…), can be used to expand iterables like an array or a string in places where zero or more arguments or elements are expected. Here is an example:Ĭonsole.log(colors) Insert One or Multiple Elements at a Specific Index Using the Spread Operator in ES6 The elements will be added before the given index after counting backwards. This will start the insertion from the end of the original array. You can also provide the first parameter as a negative value. Var deleted = colors.splice(2, 2, "Blue", "Green") This means that if you delete anything from the original array, the deleted elements can be stored in a variable. The splice() method also returns all the deleted elements as an array. Otherwise, the they would be inserted as an array. In the above example, you might have noticed that the elements to be inserted into the array need to be provided separately. The following examples should make the insertion process clear: They will be inserted one after the other consecutively. You can also insert more than one element at once. The third parameter is the element that you want to insert into the original array. The second parameter determines the number of elements that you want to delete from the original array. The first parameter determines the index at which you want to insert the new element or elements. The JavaScript Array.splice() method is used to change the contents of an array by removing existing elements and optionally adding new elements. Insert One or Multiple Elements at a Specific Index Using Array.splice() Insert One or Multiple Elements After a Specific Element in an Array.Insert One or Multiple Elements Before a Specific Element in an Array.Insert One or Multiple Elements at the End of an Array Using Array.push().

Insert One or Multiple Elements at a Specific Index Using the Spread Operator in ES6.Insert One or Multiple Elements at a Specific Index Using Array.splice().
